Programming is a fun activity that allows you to create computer programs, games, and more. To write a program, you need to use special tools and techniques. One such technique is the use of algorithms.
Algorithm is a set of instructions that must be followed in order to achieve a certain result. Algorithms are used in a variety of fields, including math, science, business, and programming.
Imagine you have a box full of lego cubes in different colors and shapes. You want to build a car out of these cubes. How are you going to do this?
Here's an example of an algorithm that can help you build a car out of Lego cubes:
- Select cubes for the base of the machine.
- Connect the selected cubes to form the base of the car with the wheels.
- Select car body cubes.
- Connect the selected cubes to make the car body.
- Place the car body on a base with wheels.
In programming, there are many different algorithms that are used to solve various problems. Here are a few examples of algorithms:
Sequential algorithm is a simple type of algorithm in which actions are performed one by one, one after another, as in a list of instructions.
For example, let's build a house in Minecraft using the Python programming language. Our algorithm would look like this:
First of all, we link our code to Minecraft so that Minecraft can understand our commands (instructions) using the import mc command.
The second step is to enable construction; this is what the world module is for, and that's what we take.
The modules contain commands that we can use from the world module. The third step is to take the BuildHome command, which builds the house, the numbers in brackets are the parameters of our house, the first three zeros are its x, y, z coordinates, followed by the dimensions: width and length 7 blocks, and height — 5, then specify the id of the block from which the house will be made, that is, its material, in this case 5 — oak boards.
So, using an algorithm of three commands, we got this house:
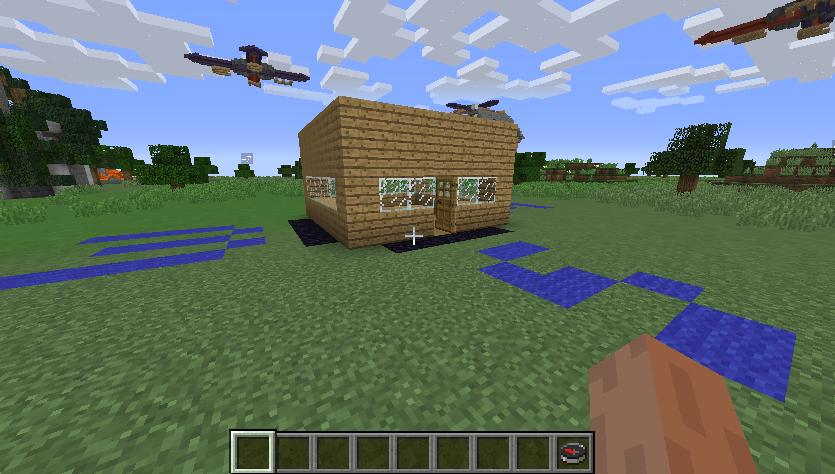
Branching algorithm is an algorithm that performs various actions depending on conditions. For example, let's write a program with the following condition: if we set the number 41, the program puts a block of gold, and if 57, then a diamond block.
The program will look like:
On line 3, we create a variable; this is a box that can store something, in our case it will store the number that we will write there.
On line 4, we write the condition using the if keyword and check whether our value is 41, if so, using the World.setBlock command, a block with id 41, that is, a block of gold, will appear at coordinates 0,0,0.
Now we have set the number 41 in the variable, let's see what we have in Minecraft:
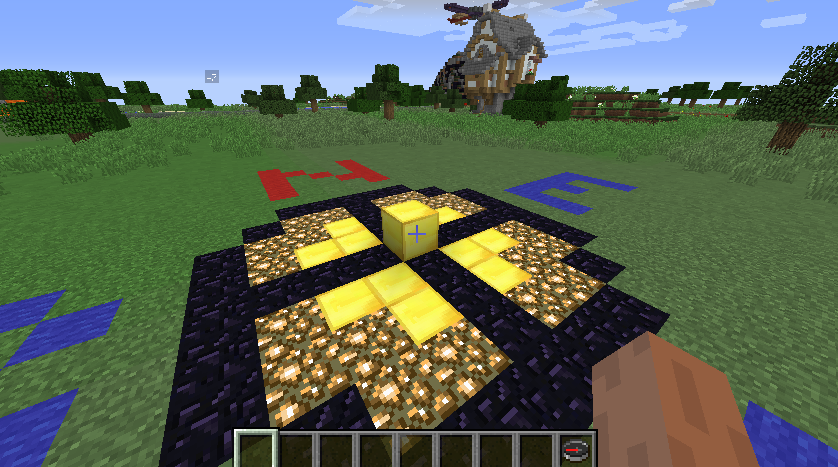
A block of gold has appeared! Now let's set another number, put 57 in the block variable, and leave everything else as it was:
In this case, the condition on line 4 is not met, because the block variable is not equal to 41, so the program will not bet gold, but will move on to the next condition — on line 6, when the block value is compared to 57, since the values are now equal, we will have a diamond block, like last time, using the SetBlock command we set it at coordinates 0.0.0, but we specify something else with id — 57, that is, a diamond block. Let's check:
.png)
This is how the branching algorithm works! It executes commands depending on conditions.
Linear algorithm is an algorithm that processes data sequentially, performing one action after another.
An example of such an algorithm would be ordinary mathematical operations. Let's write a program like this:
Here we have 3 variables: x, y, z. We put 5 in x, 10 in y, and the sum of x and y values in z. Then we used these variables as coordinates to build a 10-block lapis lazuli column.
On line 5, when we calculate the value of the variable z, we use a linear algorithm — we add the previously specified values.
Let's look at the result:
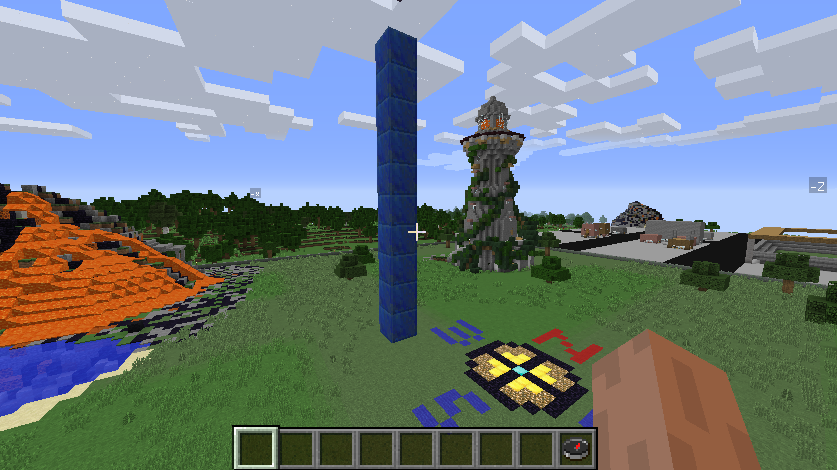
Cycle algorithm is an algorithm that performs actions repeatedly as long as a certain condition is met. For example, if we want to have not 1 house, as in the first example, but a whole village, we can easily build houses using this algorithm.
Let's write this code:
Here we create the home variable and put 0 in it, which will count the number of houses built.
Next, set the x coordinate from which the construction of the houses will begin.
This is followed by the cycle algorithm itself. Let's use a while loop that will build houses until the home variable becomes 6, so we should end up with 6 houses.
In the while loop, we write what we want to do, what commands to repeat. In this case, we repeat the construction of houses with the BuildHome command, then change the x coordinate, add the number 10 to it so that the houses are not close to each other.
Finally, add 1 to the home variable to count the built house. These commands are executed as many times as we specify in the While loop condition.
Let's see what we got:
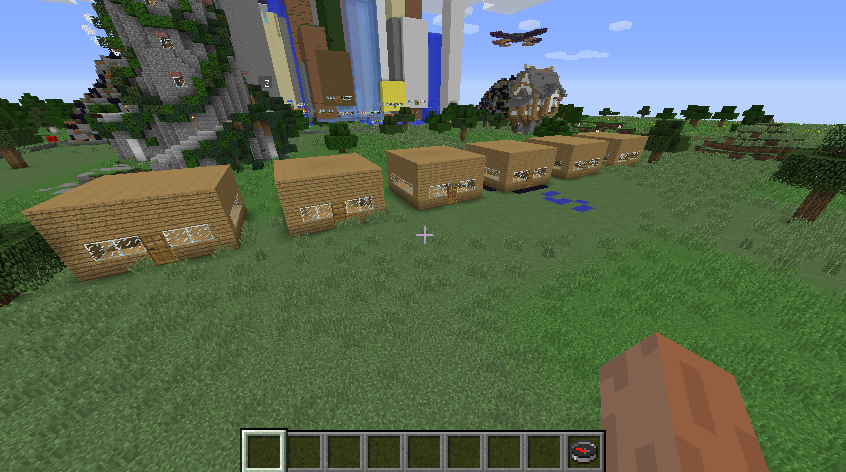
Auxiliary algorithm is an algorithm that helps another algorithm perform a specific task. It can be called several times from the main algorithm or used only once to solve a specific problem.
Let's say we want to place 10 blocks, 5 of which will be oak boards and the remaining 5 will be stone.
To put several blocks in a row, we will use the previously studied cycle algorithm — the while loop, and as an auxiliary algorithm we will use the branching algorithm — the if condition.
Let's write a program like this:
Here, using the while loop, we place blocks; inside the while loop itself, there is our auxiliary algorithm — the if condition. And the condition is this: if the x coordinate at which we place blocks is less than or equal to 5, then we will place a stone. At some point, the condition will stop being met, that is, when x becomes 6, in which case we will also continue to place blocks, but this time they will also place oak boards.
Here's what we got:
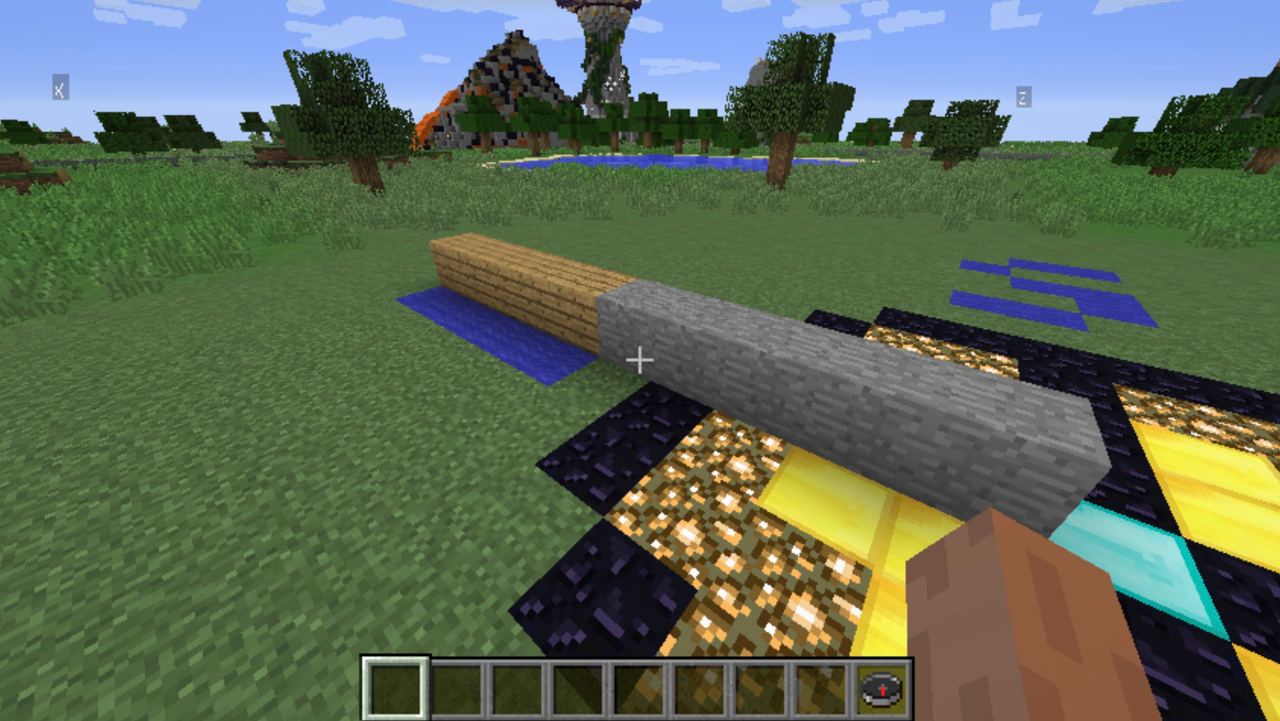
Let us sum up the results. Knowledge of algorithms is very important for programming. When you're writing a program, you need to give your computer instructions on what it should do. Algorithms are these instructions. The algorithms presented in the article are the most basic. It is necessary and useful to know them. There are many other types of algorithms, but most of them are only applicable to solving problems in a particular one of the many areas of programming.
Now you know what algorithms are in programming and what they are. If you want to learn programming and create similar and better projects, we recommend signing up for a free trial lesson on the Python programming language at our Progkids programming school for children.